Lecture 7: Pointer variables (an overview)
Objectives of this lecture
q Learn how to declare and use pointer variables
q Differentiate between a variable and a pointer to the
variable
q Be able to manipulate a variable directly (though its
name) and indirectly (through its pointer)
What is a pointer variable and how is it declared?
q Understanding pointer variables is a key to
understanding C language as pointers are used extensively by C.
q A variable is a name associated with a physical addressable
location within the computers memory.
Eg. int length, width;
float area;
length
|
width
|
area
|
???
|
???
|
???
|
1000
|
1001
|
1002
|
1003
|
1004
|
1005
|
1006
|
1007
|
|
|
|
|
|
|
|
|
q The address of a variable is called its lvalue & its associated data is called its rvalue.
q A pointer is a variable that can hold the memory
address (lvalue) of another variable (or the constant
NULL ).
q It is a legitimate data type in C whose range is the
address space of the computers memory and it can be printed using the %p
format specifier.
q Pointer variables are usually associated with a
specific type int pointer, char pointer, etc. WHY?
q A pointer variable is declared by preceeding it its
name with an asterisks *
E.g. int *x
float *y, *z
char* ch
q Note that initially these variables are not pointing
to anything. As with most variables, they are said to contain logical garbage
or simply undefined)
Assignment of values to pointer variables
q A pointer variable can be assigned a value (address of
another variable) at declaration.
E.g. int
i , *p=&i , k=5 , *r=&k;
q Note: *p = &i ; è the pointer variable p is initialized with the address of the variable
i . It does not mean a variable *p is initialized with the address of i.
q A pointer variable can also be assigned a value using
the assignment statement.
E.g.
x=&length
Y=&area
q When the address of a variable (e.g. length) is assigned to a pointer variable (x = &length), the value of the variable can be accessed in two
ways:
q Directly using variable name: e.g. length =10
q Indirectly (indirect addressing) using the dereference
operator, *, e.g. *x=20
q Note that *x is usually read as the objected being
pointed by x.
q Because *x can be used to change the value of length,
it is sometimes called an alias for length.
Pointer assignment
q Pointers of the same type can be assigned. Consider
the declarations:
int a
= 4 , *p1 =
&a ;
int b
= 20 , *p2 =
&b ;
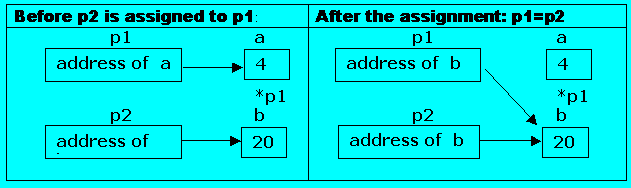
q Note: After the assignment, the variable a can only be accessed directly; because the pointer p1 no longer points to it.
q The variable b can now be
accessed directly as b, indirectly as *p1 or indirectly as
*p2.
q Two pointers are said to be equal if they point to the
same object. For example, after the
assignment p1=p2 above, p1 is equal to p2. That is, p1==p2 returns true.
Examples
1. which of the following statement is
correct. If not, why not. Assume the following declarations:
int
a, *i_pt1, *i_pt2;
float
b, *fl_pt1, *fl_pt2;
char
c, *c_pt1, *c_pt2;
#include <stdio.h>
main()
{ int count = 10, x, *ip;
ip = &count;
x = *ip;
printf("count = %d, x = %d\n", count, x);
}
#include <stdio.h>
main()
{ int i1, i2, *p1, *p2;
i1 = 5;
p1 = &i1;
i2 = *p1 / 2 + 10;
p2 = p1;
printf("i1=%d, i2=%d, *p1=%d, *p2=%d\n", i1, i2, *p1, *p2);
}